Use of Ternary Operator
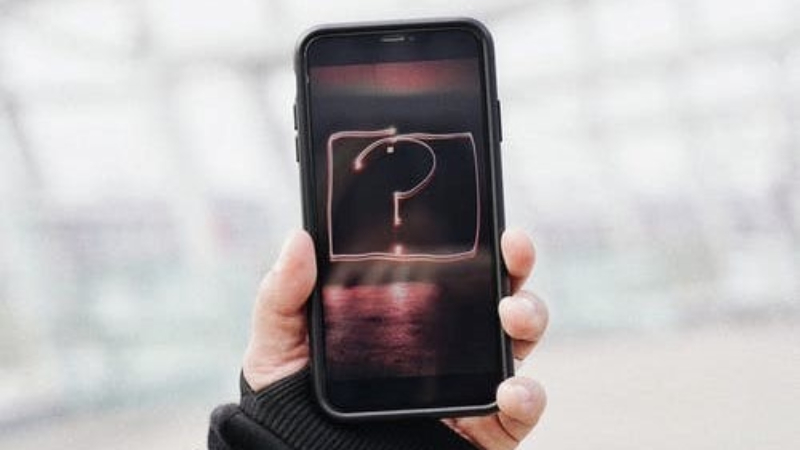
In this post we will learn about Ternary Operator in Javascript and its usage.
So we all know the classical JavaScript conditional statement if else. Below is example:
var a = 10, b = 20;
if(a > b) {
console.log("A is Greater");
}
else {
console.log("B is Greater");
}
So here the we provide an condition in if statement which if turns true then expression under the if block is executed otherwise expression under else block is executed. The same code can be written using Ternary operator provided by JavaScript
The conditional (ternary) operator is the only JavaScript operator that takes three operands.
condition ? exprIfTrue : exprIfFalse
Example:
var a = 10, b = 20;
a > b ? console.log("A is Greater") : console.log("B is Greater");
Besides false
, possible falsy expressions are: null
, NaN
, 0
, the empty string (""
), and undefined
. The ternary operator can further be used for chaining multiple conditions as follows
function example(…) {
return condition1 ? value1
: condition2 ? value2
: condition3 ? value3
: value4;
}
function example(…) {
if (condition1) { return value1; }
else if (condition2) { return value2; }
else if (condition3) { return value3; }
else { return value4; }
}
Hope you have understood the usage of Ternary Operator.
Happy Coding!