Subscribe to Keyboard Events using event methods and HostListener
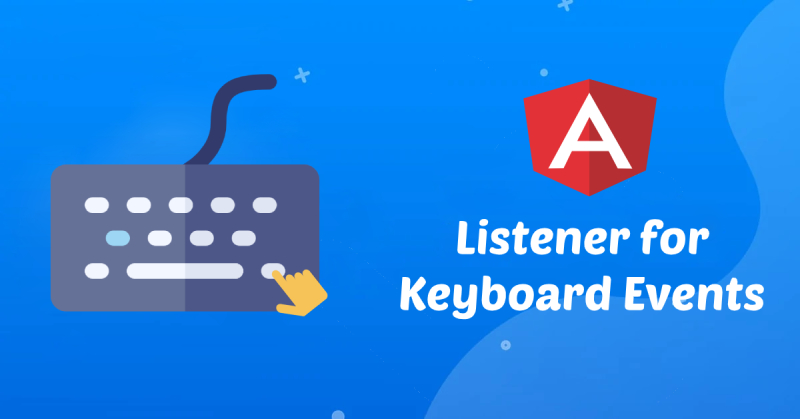
In this post we are going to learn how to subscribe to angular keyboard events like keypress, keyup etc. We all know the traditional method of onkeypress and onkeydown methods in HTML, well we can use the same in Angular too. Also Angular provides additional method known as HostListener by which we can listen to all the events placed from our application.
We can use the angular HostListener API for listening to several events like mouse events, scroll events etc, but for our purpose we will configure this method to listen to keyboard events.
Angular Keyboard Events
Below example shows how to bind to the keyup
event on an input box to get the user's input after each keystroke.
<input (keyup)="onKey($event)">
Email: <p>{{email}}</p>
export class AppComponent { 
email: String = '';
onKey(event: KeyboardEvent) {
this.email = event.target['value'];
console.log("event", event);
}
}
The $event
event payload passed to the component's event handler "onKey" has useful data which you can use for your coding logic and put conditions according to your requirements. You can console the event object and check all the properties which you get through KeyboardEvent.
Just like keyup
(also referred as ng-keyup) you can also use the keypress
(also referred as ng-keypress) and keydown
(also referred as ng-keydown) events on the input box. The series in which these events fired are like.
-
keydown
happens first -
keypress
happens second (when text is entered) -
keyup
happens last (when text input is complete).
To check this which event fires up first is hook in all three event to the same input and check the console as below
Html:
<input (keyup)="onKey($event)" (keypress)="onKey($event)" (keydown)="onKey($event)" >
Ts:
export class AppComponent {
onKey(event: KeyboardEvent) {
console.log("This is", event.type);
}
}
Output:
Angular Hostlistener
Apart from this the other method which we can use is the Angular's HostListener API which we can import and subscribe to all the KeyboardEvents occurring in our application.
import { HostListener } from '@angular/core';
export class AppComponent {
@HostListener("window:keypress", ["$event"])
handleKeyboardEvent(event: KeyboardEvent) {
console.log(event);
}
}
Here we have used "keypress" event listener to the global window object so we don't have to add event listeners from html explicitly our HostListener method does the job for us and whenever a "keypress" event takes place it will log the event object to your console. You can subscribe to other events like mouse events, scroll events etc as well.
Happy Coding!