Capitalize First Letter Javascript
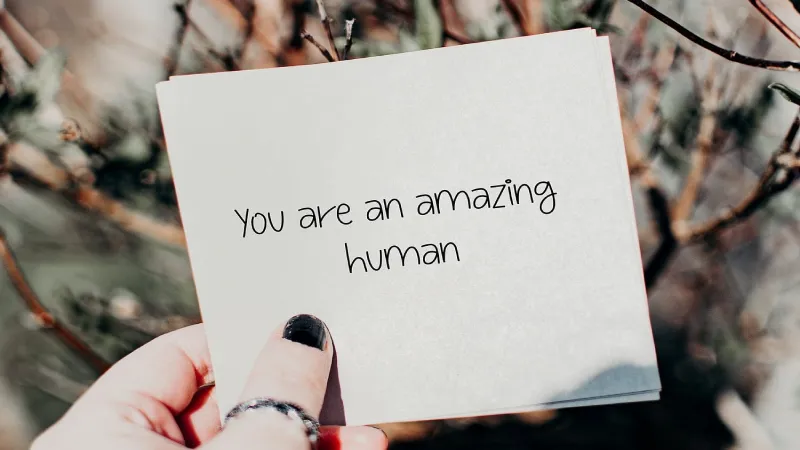
In this post we are going to learn how to capitalize first letter of string in Javascript. In Javascript it provides String.toUpperCase() method which capitalizes all letters of a string.
But what if we want to capitalize only first Letter of the String. So we will write our custom function to capitalize only first letter of the string provided.
function capitalize(str) {
if (str && typeof(str) == 'string') {
return str.charAt(0).toUpperCase() + str.slice(1);
}
else {
return null;
}
}
This can be simplified as belows:
function capitalize(str) {
return (str && typeof(str) == 'string') ? str[0].toUpperCase() + str.slice(1) : null;
}
So you can call this method and pass your string and it will return the desired output
var string = "you are an amazing human";
capitalize(string);
So here we have created a function named as capitalized which will first check the data type of given arguments is string, it will return null if its empty or not string.
We will take the index of first letter of the complete string and use String.toUpperCase() method to capitalize it and then concatenate it with rest of the string by removing out the smaller first letter from it.
Happy Coding!